Chapter 11 Check Point Questions
Section 11.2
▼11.2.1
True or false? A subclass is a subset of a superclass.
▼11.2.2
What keyword do you use to define a subclass?
▼11.2.3
What is single inheritance? What is multiple inheritance? Does Java support multiple inheritance?
Section 11.3
▼11.3.1
What is the output of running the class C in (a)? What problem arises in compiling the program in (b)?
(a) class A { public A() { System.out.println("A's no-arg constructor is invoked"); } } class B extends A { } public class C { public static void main(String[] args) { B b = new B(); } } (b) class A { public A(int x) { } } class B extends A { public B() { } } public class C { public static void main(String[] args) { B b = new B(); } }
▼11.3.2
How does a subclass invoke its superclass's constructor?
▼11.3.3
True or false? When invoking a constructor from a subclass, its superclass's no-arg constructor is always invoked.
Section 11.4
▼11.4.1
True or false? You can override a private method defined in a superclass.
▼11.4.2
True or false? You can override a static method defined in a superclass.
▼11.4.3
How do you explicitly invoke a superclass's constructor from a subclass?
▼11.4.4
How do you invoke an overridden superclass method from a subclass?
Section 11.5
▼11.5.1
Identify the problems in the following code:
1 public class Circle { 2 private double radius; 3 4 public Circle(double radius) { 5 radius = radius; 6 } 7 8 public double getRadius() { 9 return radius; 10 } 11 12 public double getArea() { 13 return radius * radius * Math.PI; 14 } 15 } 16 17 class B extends Circle { 18 private double length; 19 20 B(double radius, double length) { 21 Circle(radius); 22 length = length; 23 } 24 25 @Override 26 public double getArea() { 27 return getArea() * length; 28 } 29 }
▼11.5.2
Explain the difference between method overloading and method overriding.
▼11.5.3
If a method in a subclass has the same signature as a method in its superclass with the same return type, is the method overridden or overloaded?
▼11.5.4
If a method in a subclass has the same signature as a method in its superclass with a different return type, will this be a problem?
▼11.5.5
If a method in a subclass has the same name as a method in its superclass with different parameter types, is the method overridden or overloaded?
▼11.5.6
What is the benefit of using the @Override annotation?
Section 11.7
▼11.7.1
What are the three pillars of object-oriented programming? What is polymorphism?
Section 11.8
▼11.8.1
What is dynamic binding?
▼11.8.2
Describe the difference between method matching and method binding.
▼11.8.3
Can you assign new int[50], new Integer[50], new String[50], or new Object[50], into a variable of Object[] type?
▼11.8.4
What is wrong in the following code?
1 public class Test { 2 public static void main(String[] args) { 3 Integer[] list1 = {12, 24, 55, 1}; 4 Double[] list2 = {12.4, 24.0, 55.2, 1.0}; 5 int[] list3 = {1, 2, 3}; 6 printArray(list1); 7 printArray(list2); 8 printArray(list3); 9 } 10 11 public static void printArray(Object[] list) { 12 for (Object o: list) 13 System.out.print(o + " "); 14 System.out.println(); 15 } 16 }
▼11.8.5
Show the output of the following code:
(a) public class Test { public static void main(String[] args) { new Person().printPerson(); new Student().printPerson(); } } class Student extends Person { @Override public String getInfo() { return "Student"; } } class Person { public String getInfo() { return "Person"; } public void printPerson() { System.out.println(getInfo()); } } (b) public class Test { public static void main(String[] args) { new Person().printPerson(); new Student().printPerson(); } } class Student extends Person { private String getInfo() { return "Student"; } } class Person { private String getInfo() { return "Person"; } public void printPerson() { System.out.println(getInfo()); } }
▼11.8.6
Show the output of following program:
1 public class Test { 2 public static void main(String[] args) { 3 A a = new A(3); 4 } 5 } 6 7 class A extends B { 8 public A(int t) { 9 System.out.println("A's constructor is invoked"); 10 } 11 } 12 13 class B { 14 public B() { 15 System.out.println("B's constructor is invoked"); 16 } 17 }Is the no-arg constructor of Object invoked when new A(3) is invoked?
▼11.8.7
Show the output of following program:
public class Test { public static void main(String[] args) { new A(); new B(); } } class A { int i = 7; public A() { setI(20); System.out.println("i from A is " + i); } public void setI(int i) { this.i = 2 * i; } } class B extends A { public B() { System.out.println("i from B is " + i); } public void setI(int i) { this.i = 3 * i; } }
▼11.8.8
Show the output of following program:
public class Test { public static void main(String[] args) { Apple a = new Apple(); System.out.println(a); System.out.println("---------------"); GoldenDelicious g = new GoldenDelicious(7); System.out.println(g); System.out.println("---------------"); Apple c = new GoldenDelicious(8); System.out.println(c); } } class Apple { double weight; public Apple() { this(1); System.out.println("Apple no-arg constructor"); } public Apple(double weight) { this.weight = weight; System.out.println("Apple constructor with weight"); } @Override public String toString() { return "Apple: " + weight; } } class GoldenDelicious extends Apple { public GoldenDelicious() { this(5); System.out.println("GoldenDelicious non-arg constructor"); } public GoldenDelicious(double weight) { super(weight); this.weight = weight; System.out.println("GoldenDelicious constructor with weight"); } @Override public String toString() { return "GoldenDelicious: " + weight; } }
Section 11.9
▼11.9.1
Indicate true or false for the following statements:
(a) You can always successfully cast an instance of a subclass to a superclass.
(b) You can always successfully cast an instance of a superclass to a subclass.
(a) You can always successfully cast an instance of a subclass to a superclass.
(b) You can always successfully cast an instance of a superclass to a subclass.
▼11.9.2
For the GeometricObject and Circle classes in Listings 11.1 and 11.2, answer the following questions:
a. Assume that circle and object are created as follows:
a. Assume that circle and object are created as follows:
Circle circle = new Circle(1); GeometricObject object = new GeometricObject();Are the following Boolean expressions true or false?
(circle instanceof GeometricObject) (object instanceof GeometricObject) (circle instanceof Circle) (object instanceof Circle)b. Can the following statements be compiled?
Circle circle = new Circle(5); GeometricObject object = circle;c. Can the following statements be compiled?
GeometricObject object = new GeometricObject();
Circle circle = (Circle)object;
▼11.9.3
Suppose that Fruit, Apple, Orange, GoldenDelicious, and McIntosh are defined in the following inheritance hierarchy:
Assume that the following code is given:
a. Is fruit instanceof Fruit?
b. Is fruit instanceof Orange?
c. Is fruit instanceof Apple?
d. Is fruit instanceof GoldenDelicious?
e. Is fruit instanceof McIntosh?
f. Is orange instanceof Orange?
g. Is orange instanceof Fruit?
h. Is orange instanceof Apple?
i. Suppose the method makeAppleCider is defined in the Apple class. Can fruit invoke this method? Can orange invoke this method?
j. Suppose the method makeOrangeJuice is defined in the Orange class. Can orange invoke this method? Can fruit invoke this method?
k. Is the statement Orange p = new Apple() legal?
l. Is the statement McIntosh p = new Apple() legal?
m. Is the statement Apple p = new McIntosh() legal?
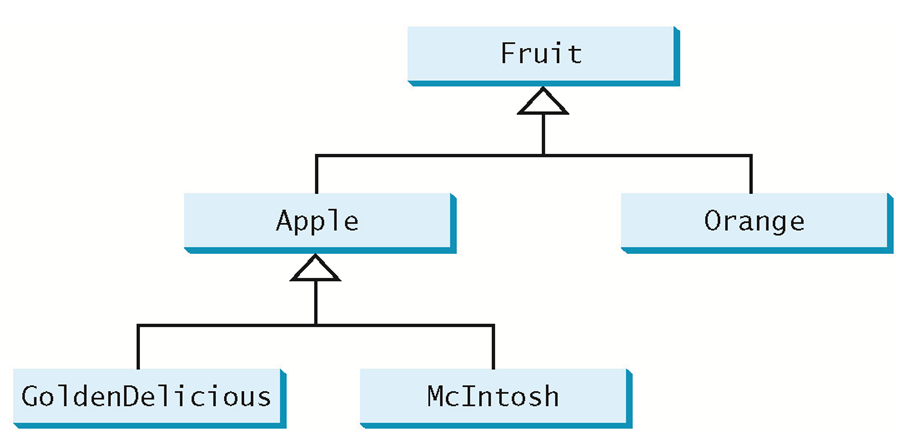
Assume that the following code is given:
Fruit fruit = new GoldenDelicious(); Orange orange = new Orange();Answer the following questions:
a. Is fruit instanceof Fruit?
b. Is fruit instanceof Orange?
c. Is fruit instanceof Apple?
d. Is fruit instanceof GoldenDelicious?
e. Is fruit instanceof McIntosh?
f. Is orange instanceof Orange?
g. Is orange instanceof Fruit?
h. Is orange instanceof Apple?
i. Suppose the method makeAppleCider is defined in the Apple class. Can fruit invoke this method? Can orange invoke this method?
j. Suppose the method makeOrangeJuice is defined in the Orange class. Can orange invoke this method? Can fruit invoke this method?
k. Is the statement Orange p = new Apple() legal?
l. Is the statement McIntosh p = new Apple() legal?
m. Is the statement Apple p = new McIntosh() legal?
▼11.9.4
What is wrong in the following code?
1 public class Test { 2 public static void main(String[] args) { 3 Object fruit = new Fruit(); 4 Object apple = (Apple)fruit; 5 } 6 } 7 8 class Apple extends Fruit { 9 } 10 11 class Fruit { 12 }
Section 11.10
▼11.10.1
Does every object have a toString method and an equals method?
Where do they come from? How are they used? Is it appropriate to override these methods?
▼11.10.2
When overriding the equals method, a common mistake is mistyping its signature in the subclass. For example, the equals method is incorrectly written as equals(Circle circle), as shown in (a) in following the code; instead, it should be equals(Object circle), as shown in (b). Show the output of running class Test with the Circle class in (a) and in (b), respectively.
the Circle class in (a) and (b), respectively?
Suppose that circle1.equals(circle2) is replaced by circle1.equals("Binding"), what would happen to run Test using the Circle class in (a) and (b), respectively? Reimplement the equals method in (b) to avoid a runtime error when comparing circle with a non-circle object.
public class Test { public static void main(String[] args) { Object circle1 = new Circle(); Object circle2 = new Circle(); System.out.println(circle1.equals(circle2)); } } (a) class Circle { double radius; public boolean equals(Circle circle) { return this.radius == circle.radius; } } (b) class Circle { double radius; public boolean equals(Object o) { return this.radius == ((Circle)o).radius; } }If Object is replaced by Circle in the Test class, what would be the output to run Test using
the Circle class in (a) and (b), respectively?
Suppose that circle1.equals(circle2) is replaced by circle1.equals("Binding"), what would happen to run Test using the Circle class in (a) and (b), respectively? Reimplement the equals method in (b) to avoid a runtime error when comparing circle with a non-circle object.
Section 11.11
▼11.11.1
How do you do the following?
a. Create an ArrayList for storing double values?
b. Append an object to a list?
c. Insert an object at the beginning of a list?
d. Find the number of objects in a list?
e. Remove a given object from a list?
f. Remove the last object from the list?
g. Check whether a given object is in a list?
h. Retrieve an object at a specified index from a list?
a. Create an ArrayList for storing double values?
b. Append an object to a list?
c. Insert an object at the beginning of a list?
d. Find the number of objects in a list?
e. Remove a given object from a list?
f. Remove the last object from the list?
g. Check whether a given object is in a list?
h. Retrieve an object at a specified index from a list?
▼11.11.2
Identify the errors in the following code.
ArrayList<String> list = new ArrayList<>(); list.add("Denver"); list.add("Austin"); list.add(new java.util.Date()); String city = list.get(0); list.set(3, "Dallas"); System.out.println(list.get(3));
▼11.11.3
Suppose the ArrayList list contains {"Dallas", "Dallas", "Houston", "Dallas"}. What is the list after invoking list.remove("Dallas") one time? Does the following code correctly remove all elements with value "Dallas" from the list? If not, correct the code.
for (int i = 0; i < list.size(); i++) list.remove("Dallas");
▼11.11.4
Explain why the following code displays [1, 3] rather than [2, 3].
ArrayList<Integer> list = new ArrayList<>(); list.add(1); list.add(2); list.add(3); list.remove(1); System.out.println(list);How do you remove integer value 3 from the list?
▼11.11.5
Explain why the following code is wrong.
ArrayList<Double> list = new ArrayList<>(); list.add(1);
Section 11.12
▼11.12.1
Correct errors in the following statements:
int[] array = {3, 5, 95, 4, 15, 34, 3, 6, 5}; ArrayList<Integer> list = new ArrayList<>(Arrays.asList(array));
▼11.12.2
Correct errors in the following statements:
int[] array = {3, 5, 95, 4, 15, 34, 3, 6, 5}; System.out.println(java.util.Collections.max(array));
Section 11.13
▼11.13.1
Write statements that create a MyStack and add number 11 to the stack.
Section 11.14
▼11.14.1
What modifier should you use on a class so that a class in the same package can access it, but a class in a different package cannot access it?
▼11.14.2
What modifier should you use so that a class in a different package cannot access the class, but its subclasses in any package can access it?
▼11.14.3
In the following code, the classes A and B are in the same package.
If the question marks in (a) are replaced by blanks, can class B be compiled? If the question marks are replaced by private, can class B be compiled? If the question marks are replaced by protected, can class B be compiled?
(a) package p1; public class A { ? int i; ? void m() { ... } } (b) package p1; public class B extends A { public void m1(String[] args) { System.out.println(i); m(); } }
▼11.14.4
In the following code, the classes A and B are in different packages. If the question marks in (a) are replaced by blanks, can class B be compiled? If the question marks are replaced by private, can class B be compiled? If the question marks are replaced by protected, can class B be compiled?
(a) package p1; public class A { ? int i; ? void m() { ... } } (b) package p2; public class B extends A { public void m1(String[] args) { System.out.println(i); m(); } }
▼11.14.5
In the following code, the classes A, B, and Main are in the same package.
Can the Main class be compiled?
class A { protected void m() { } } class B extends A { } class Main { public void p() { B b = new B(); b.m(); } }
Section 11.15
▼11.15.1
How do you prevent a class from being extended? How do you prevent a method from being overridden?
▼11.15.2
Indicate true or false for the following statements:
a. A protected datum or method can be accessed by any class in the same package.
b. A protected datum or method can be accessed by any class in different packages.
c. A protected datum or method can be accessed by its subclasses in any package.
d. A final class can have instances.
e. A final class can be extended.
f. A final method can be overridden.
a. A protected datum or method can be accessed by any class in the same package.
b. A protected datum or method can be accessed by any class in different packages.
c. A protected datum or method can be accessed by its subclasses in any package.
d. A final class can have instances.
e. A final class can be extended.
f. A final method can be overridden.